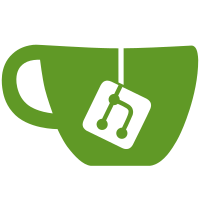
Also move static function prototypes out of compton.h. Seems like the previous developers didn't know what header files are for. Seems to have bugs after the split.
96 lines
2.3 KiB
C
96 lines
2.3 KiB
C
#include <X11/Xlib.h>
|
|
|
|
#include "common.h"
|
|
#include "x.h"
|
|
|
|
/**
|
|
* Get a specific attribute of a window.
|
|
*
|
|
* Returns a blank structure if the returned type and format does not
|
|
* match the requested type and format.
|
|
*
|
|
* @param ps current session
|
|
* @param w window
|
|
* @param atom atom of attribute to fetch
|
|
* @param length length to read
|
|
* @param rtype atom of the requested type
|
|
* @param rformat requested format
|
|
* @return a <code>winprop_t</code> structure containing the attribute
|
|
* and number of items. A blank one on failure.
|
|
*/
|
|
winprop_t
|
|
wid_get_prop_adv(const session_t *ps, Window w, Atom atom, long offset,
|
|
long length, Atom rtype, int rformat) {
|
|
Atom type = None;
|
|
int format = 0;
|
|
unsigned long nitems = 0, after = 0;
|
|
unsigned char *data = NULL;
|
|
|
|
if (Success == XGetWindowProperty(ps->dpy, w, atom, offset, length,
|
|
False, rtype, &type, &format, &nitems, &after, &data)
|
|
&& nitems && (AnyPropertyType == type || type == rtype)
|
|
&& (!rformat || format == rformat)
|
|
&& (8 == format || 16 == format || 32 == format)) {
|
|
return (winprop_t) {
|
|
.data.p8 = data,
|
|
.nitems = nitems,
|
|
.type = type,
|
|
.format = format,
|
|
};
|
|
}
|
|
|
|
cxfree(data);
|
|
|
|
return (winprop_t) {
|
|
.data.p8 = NULL,
|
|
.nitems = 0,
|
|
.type = AnyPropertyType,
|
|
.format = 0
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Get the value of a type-<code>Window</code> property of a window.
|
|
*
|
|
* @return the value if successful, 0 otherwise
|
|
*/
|
|
Window
|
|
wid_get_prop_window(session_t *ps, Window wid, Atom aprop) {
|
|
// Get the attribute
|
|
Window p = None;
|
|
winprop_t prop = wid_get_prop(ps, wid, aprop, 1L, XA_WINDOW, 32);
|
|
|
|
// Return it
|
|
if (prop.nitems) {
|
|
p = *prop.data.p32;
|
|
}
|
|
|
|
free_winprop(&prop);
|
|
|
|
return p;
|
|
}
|
|
|
|
/**
|
|
* Get the value of a text property of a window.
|
|
*/
|
|
bool wid_get_text_prop(session_t *ps, Window wid, Atom prop,
|
|
char ***pstrlst, int *pnstr) {
|
|
XTextProperty text_prop = { NULL, None, 0, 0 };
|
|
|
|
if (!(XGetTextProperty(ps->dpy, wid, &text_prop, prop) && text_prop.value))
|
|
return false;
|
|
|
|
if (Success !=
|
|
XmbTextPropertyToTextList(ps->dpy, &text_prop, pstrlst, pnstr)
|
|
|| !*pnstr) {
|
|
*pnstr = 0;
|
|
if (*pstrlst)
|
|
XFreeStringList(*pstrlst);
|
|
cxfree(text_prop.value);
|
|
return false;
|
|
}
|
|
|
|
cxfree(text_prop.value);
|
|
return true;
|
|
}
|